Version: 1.1.0
Execution Table
The following table details the execution of ServerRpc
and ClientRpc
functions:
Function | Server | Client | Host (Server+Client) |
---|---|---|---|
ServerRpc Send | |||
ServerRpc Execute | |||
ClientRpc Send | |||
ClientRpc Execute |
An RPC function typically doesn't execute its body immediately since the function call is a stand-in for a network transmission. Since the host is both a client and a server, local RPCs targeting the host-server or host-client are invoked immediately. As such, avoid nesting RPCs when running in host mode as a ServerRpc method that invokes a ClientRpc method that invokes the same ServerRpc method (and repeat...) can cause a stack overflow.
Structure of a typical ServerRpc
:
[ServerRpc]
void MyServerRpc(int somenumber, string somestring)
{
// Network Send Block (framework-code)
// Network Return Block (framework-code)
// RPC Method Body (user-code)
}
Pseudo-code sample of a ServerRpc
:
[ServerRpc]
void MyServerRpc(int somenumber, string somestring)
{
// --- begin: injected framework-code
if (NetworkSend())
{
// this block will be executed if:
// - called from user-code on client
// - called from user-code on host
var writer = NetworkCreateWriter();
writer.WriteInt32(1234567890); // RPC method signature hash
writer.WriteInt32(somenumber);
writer.WriteChars(somestring);
NetworkSendRpc(writer);
}
if (NetworkReturn())
{
// this block will be executed if:
// - called from user-code
// - called from framework-code on client
return;
}
// --- end: injected framework-code
print($"MyServerRpc: {somenumber}");
}


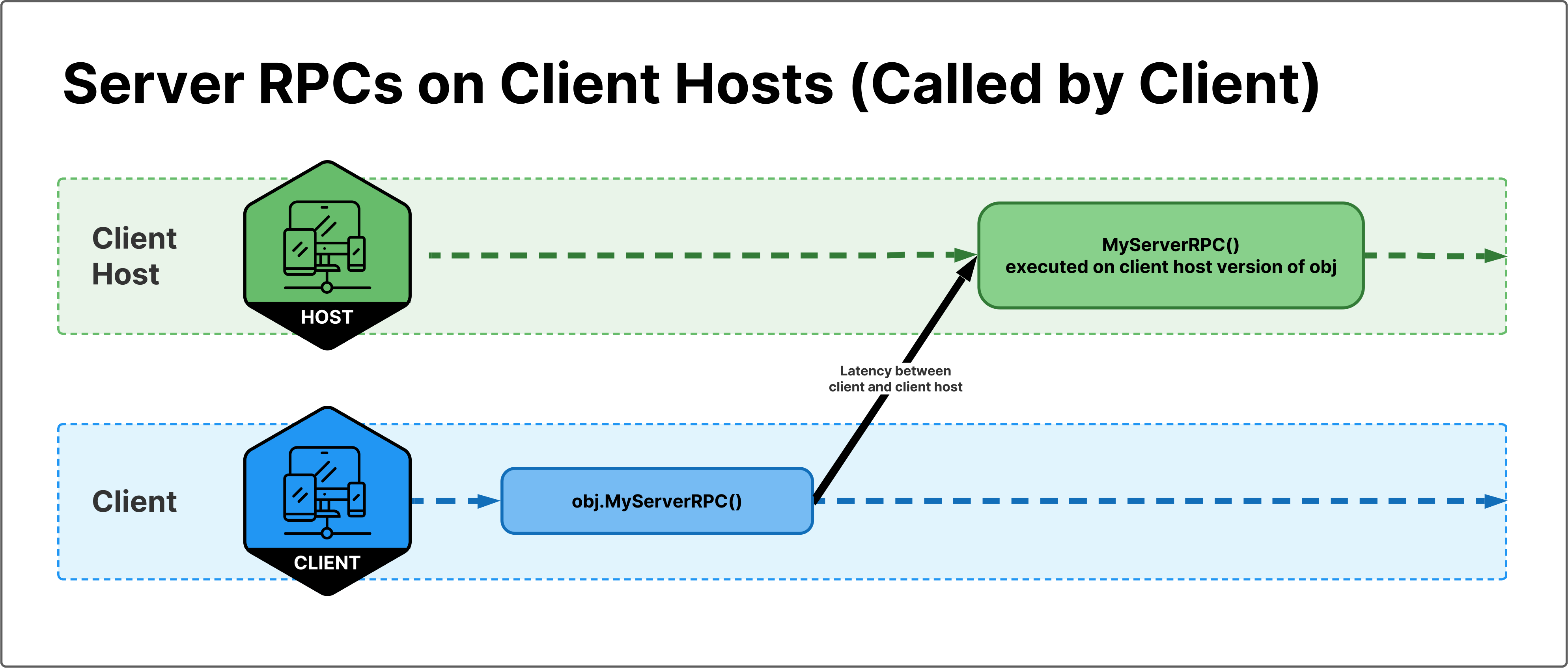
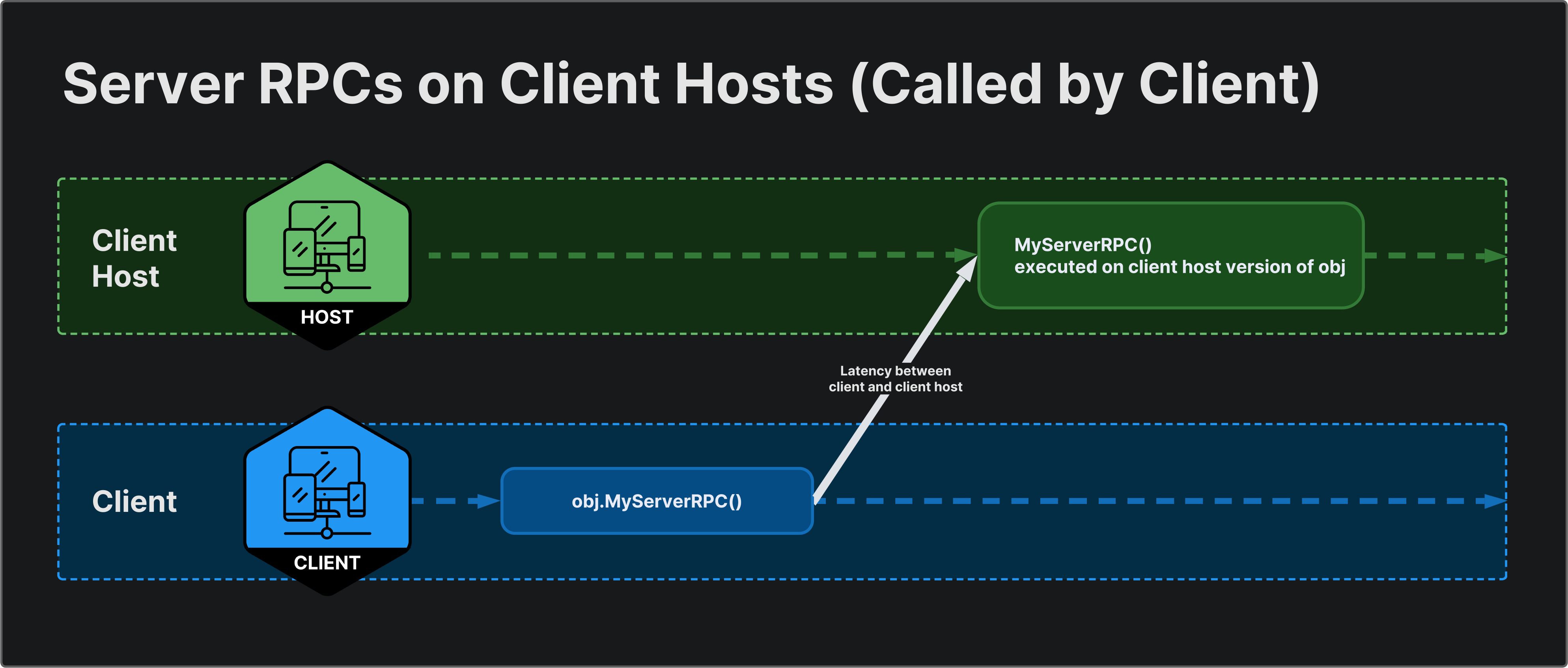
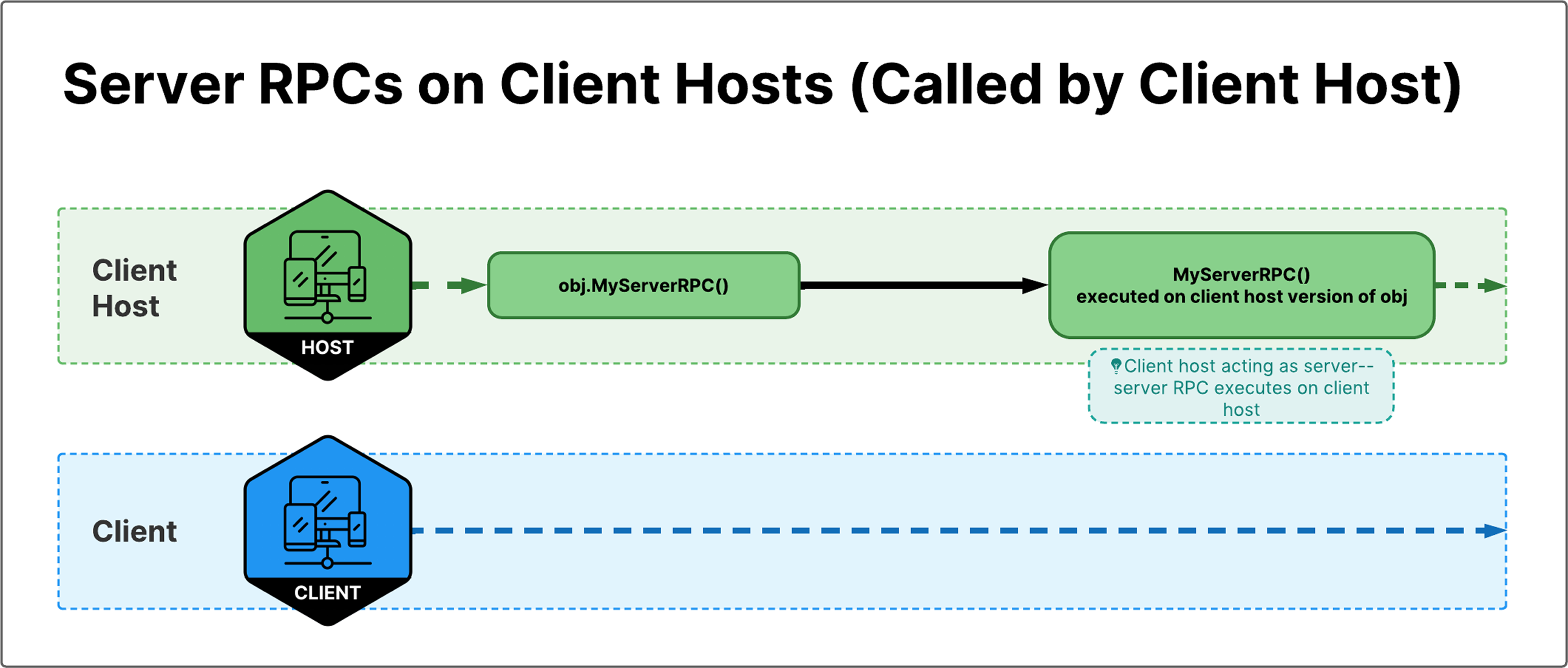

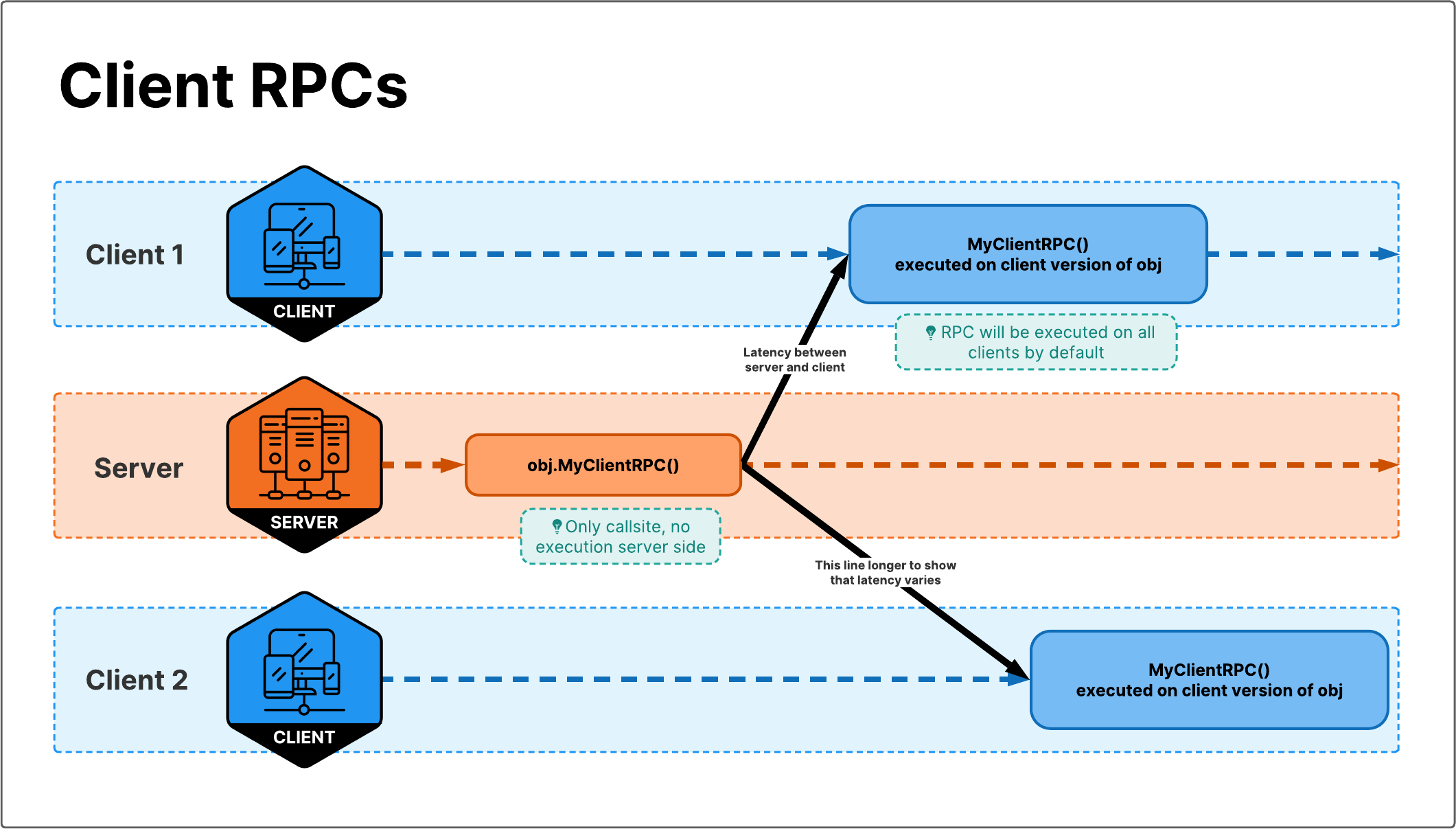
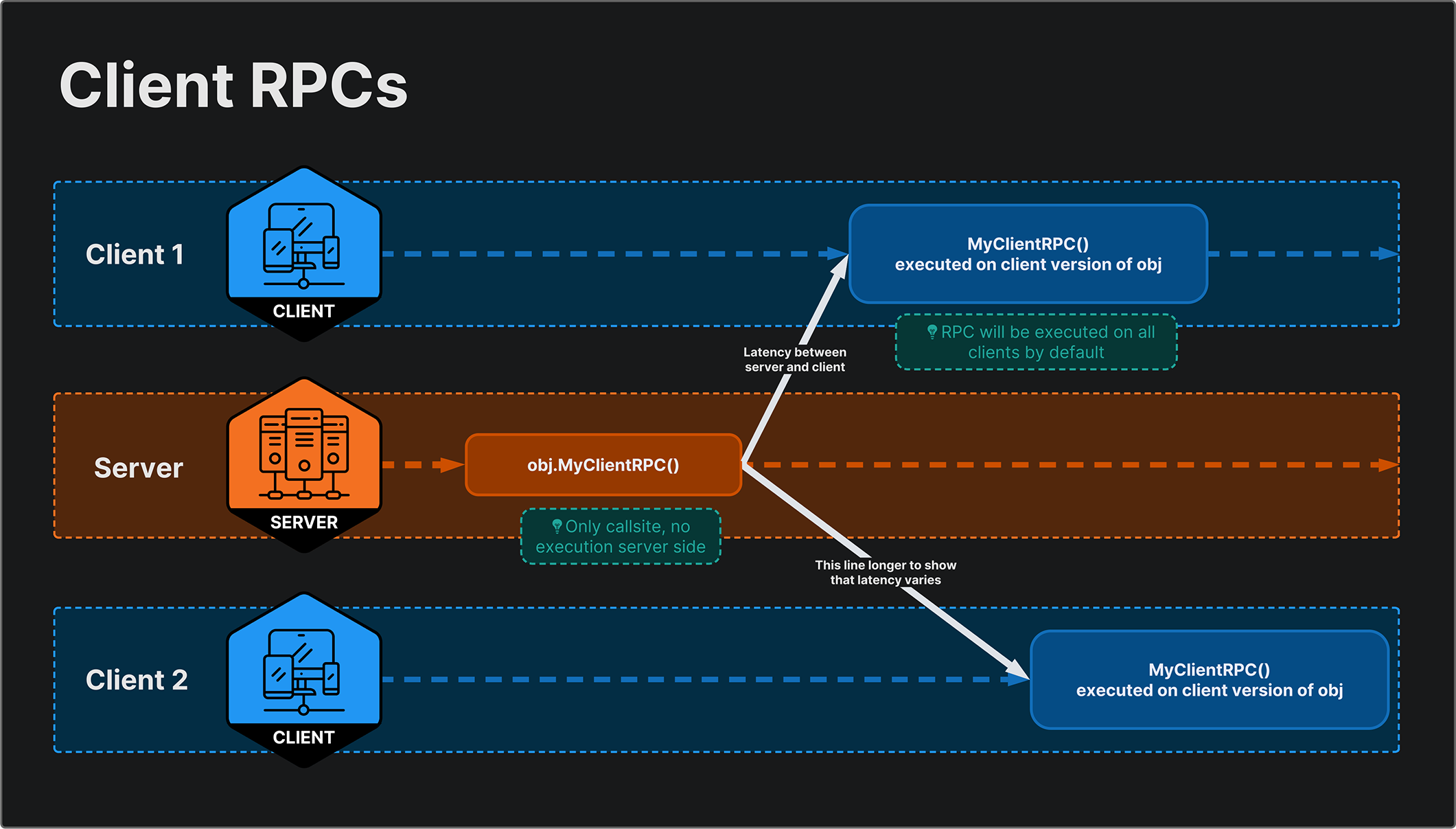

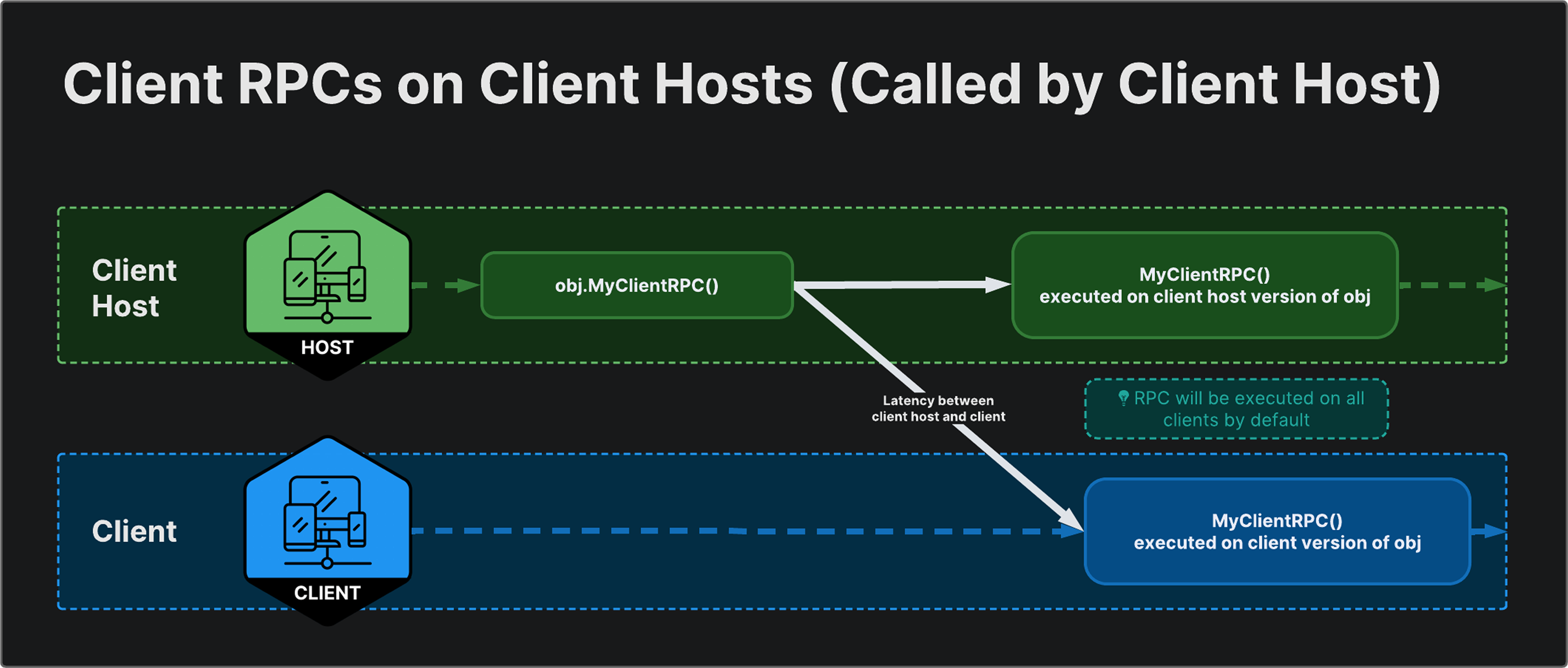